Functions Lab | Part I #
In this lab, we will learn how to create and call functions. Functions are blocks of code that are reusable throughout a program. You’ve already encountered functions such as print()
, which is a function built into Python.
[0] Set up #
๐ป Start by going into the unit folder.cd ~/desktop/making_with_code/unit00_drawing/
git clone https://github.com/the-isf-academy/lab_functions1.git
cd lab_functions1
๐ป Enter the Poetry Shell to start the lab. As a reminder, we will run this command at the start of each lab, but only when we are inside a lab folder.
poetry shell
๐พ ๐ฌ Exiting the poetry shellWhen you want to exit the shell, you can type
exit
or^D
๐ป Take a look at the files inside with:
ls
triangle.py
hexagon.py
icecream.py
[1] What is a function? #
Before we can talk about functions, we need to talk about code blocks. A code block is one or more lines of code. Here’s one:
forward(side_length)
left(120)
Sometimes we decide how many times a code block should run, or whether it should run at all. Consider this code, which will draw a triangle:
for each_item in range(3):
forward(side_length)
left(120)
We have the same code block, but now it’s indented under the loop for each_item in range(3):
. This means
the code block should run once for each item in the range.
Often times we want to reuse a code block in different sections of a program. Functions
allow for code reuse without needing to copy and paste code blocks. Here is a code block with a function definition and a function call:
|
|
A few things to note:
line 1
def
defines a new function calleddraw_triangle()
- it takes one parameter, or argument, called
side_length
line 4
side_length
is used as a variable- you can use a function’s parameters inside the function as variables
line 7
draw_triangle(200)
calls the function with aside_length
of200
- Once you have written a function, you must call it to use it.
๐พ ๐ฌFunctions make your code simpler. Instead of writing all the code for a triangle over and over, you can get it right once and put it in a function.
Once you have written a function, you must call it. Calling a function tells Python to run that block of code. Remember, order matters. Code is read from top to bottom. You cannot call a function before it has been written.
Editing a Function #
๐ป Let's start by running
triangle.py
python triangle.py
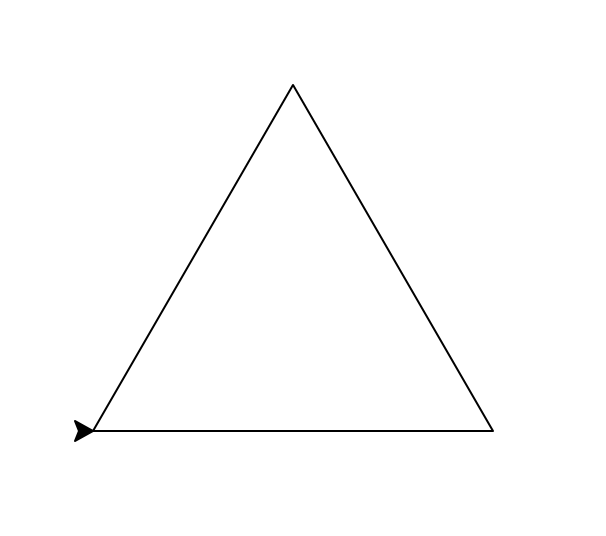
code triangle.py
Notice how it calls the draw_triangle()
function with a side_length
of 200
.
๐ป Experiment by editing the parameter and then re-running the program.
For example, change 200
to 500
.
Writing a Function #
Now that you’ve had some practice editing a function, let’s write a new one.
๐ป Open the file:
hexagon.py
code hexagon.py
๐ป Write a function that draws a hexagon with any side_length. You will need to:
- Write the function
draw_hexagon()
.- It should have one parameter called
side_length
- It should have one parameter called
- Call the function
draw_hexagon()
below the function definition.- It should be able to be called with any number for the
side_length
.
- It should be able to be called with any number for the
Your finished drawing should look something like this:
[2] Combining functions #
When you want to draw something fancy, you need to break it down into smaller steps. Then you can write functions to do the smaller steps, and write more functions to combine small steps into bigger steps. This concept is called decomposition.
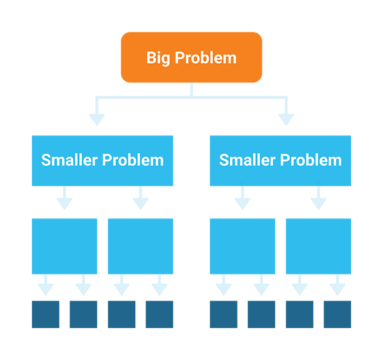
โ๏ธ If we want to draw an ice cream cone with scoops of ice cream, we can break it down into steps:
- Draw an ice cream cone:
cone(side_length)
- Draw a scoop of ice cream:
scoop(num_scoops)

Draw Ice Cream #
๐ป Open the file:
code icecream.py
. As you can see, the function definitions are already provided. You just need to call the functions:
scoop(num_scoops)
cone(size_length)
๐ป Complete the ice cream drawing by calling the functions to draw an ice cream cone.
You need to use other turtle functions and experiment with the parameters to align the scoop()
and the cone()
perfectly. A few useful turtle functions include, but not limited to:
forward()
backward()
right()
left()
penup()
pendown()
goto()
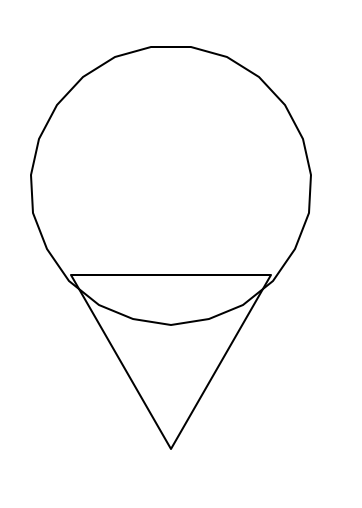
๐ป
Now, add in color by adding a second parameter to scoop()
and cone()
.
Note: a variable cannot be the same name as a function. We suggest using the parameters
scoop_color
andcone_color
.
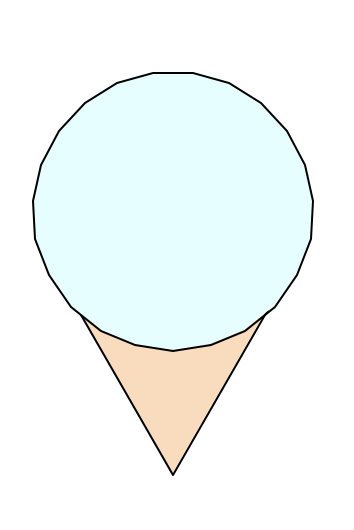
๐พ ๐ฌ FYI: How to Fill a Shape with Color and More!๐ป To fill your turtle drawing with color follow the below format. You must call
begin_fill()
before the shape has been drawn andend_fill()
after.circle_color = "blue" fillcolor(circle_color) #Tells the turtle to set the fill color begin_fill() #Tells the turtle to start the color fill circle(200) end_fill() #Tells the turtle to stop the color fill
๐ For a wide range of color options look at this chart.
[3] Deliverables #
โกโจโ๏ธ Once you’ve successfully completed the square pattern be sure to fill out this Google form.
โ๏ธ Add a screenshot of a code snippet to your
CS9 Lab Code Log
in your Google Drive. Add a comment either asking a question about your code OR describing a piece of code you are proud of.
[4] Extension: Ice Cream Parlor #
Number of Scoops #
Right now, you can only have 1 scoop of ice cream ๐ข. But, it’s way more fun to have multiple scoops of ice cream!
๐ป Add another parameter to scoop()
called num_scoops
. This should draw any number of scoops on top of each other.
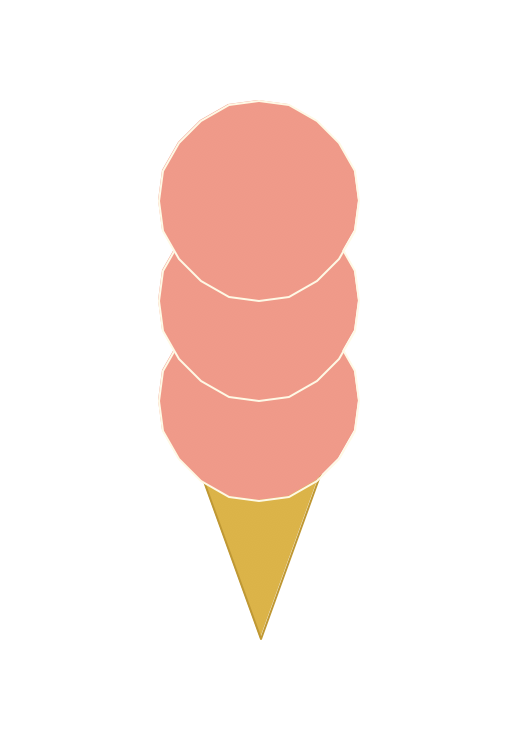
Sprinkles #
What’s even more fun than multiple scoops, sprinkles!
๐ป Write a new function, sprinkles()
that takes, flavor
as a parameter. It should randomly place sprinkles on the scoops of ice cream.
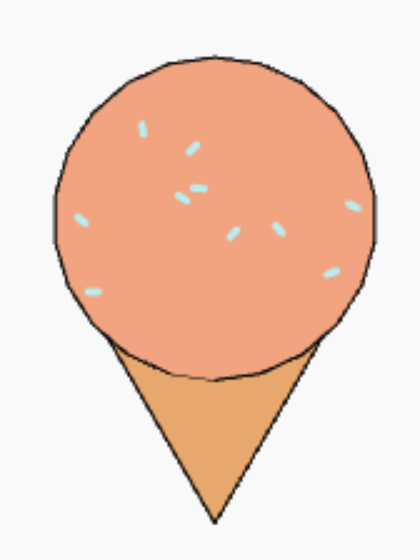
For random integers:
from random import randint
randint(0,100)
User Input #
Now that we have colors and number of scoops, let’s add in user input!
๐ป Expand the functionality of this program to simulate an ice cream parlor. The user should be able to choose the flavor of the ice-cream and the number of scoops.
๐พ ๐ฌ HintsConsider the following:
- How will you include user input?
- How will you translate the flavor “chocolate” to the color “brown?
Here is an example interaction:
python ice_cream.py
--- Welcome to the ISF ice cream parlor ---
What flavor would you like?
> Select a flavor (chocolate, strawberry, vanilla): chocolate
How many scoops would you like?
> Select number of scoops (max 3): 3e
Do you want prinkles?
> Enter yes or no: no
--- Enjoy your ice cream! Please come again! ---
[Press any key to exit]
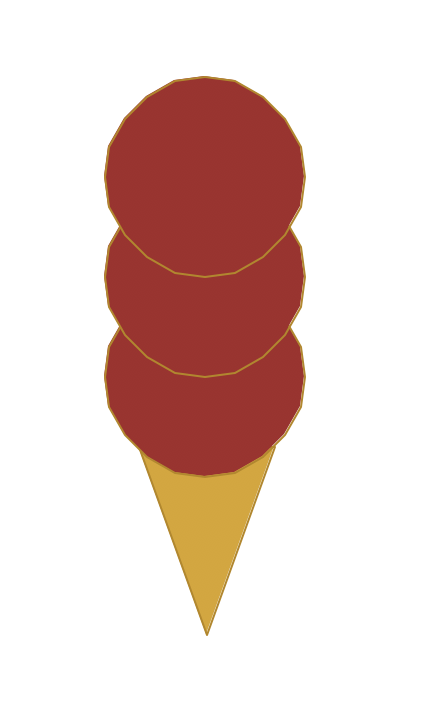