Conditionals #
In this lab, we’ll introduce a new concept: conditionals. This allows change the behavior of our code based on context.
[0] Conditional behavior #
There are many situations where you change your behavior based on the environment. For example, if it is raining outside, you wear rain boots. Otherwise, you might wear a different kind of shoe like tennis shoes.
You probably have even more complicated environmental responses as well.
For example, if you are ordering bubble tea, your order might go like this:
- If the shop has taro,
- you get taro in your tea
- If the shop doesn’t have taro but has pearl
- you get pearl in your tea
- Finally, in the case that the shop doesn’t have taro or pearl
- you don’t order any bubble tea.
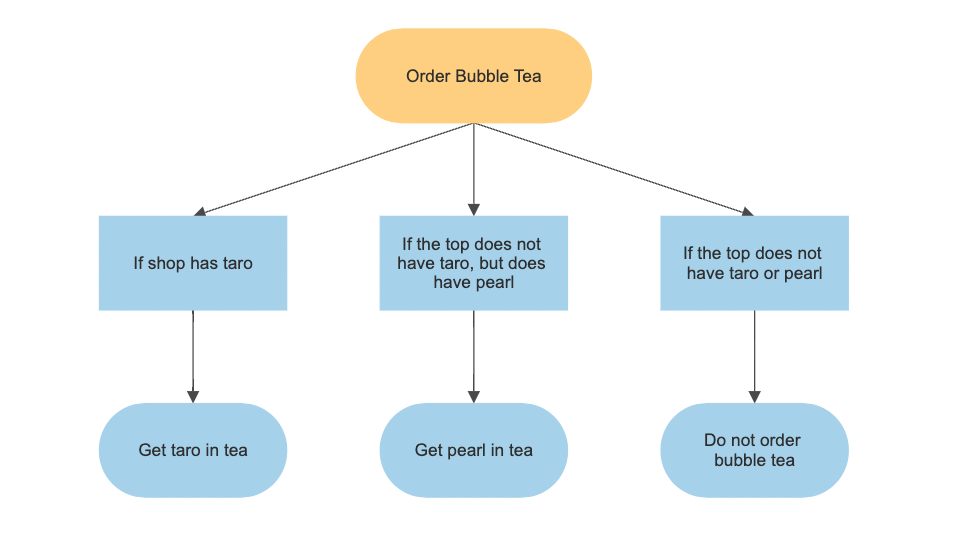
In computer science, we call this kind of behavior conditional
: your code runs only in the case that some condition is satisfied.
[1] Set up #
๐ป
Start by going into your unit00_drawing
folder.
cd ~/desktop/making_with_code/unit00_drawing/
git clone https://github.com/the-isf-academy/lab_conditionals.git
cd lab_conditionals
๐ป Enter the Poetry Shell to start the lab. As a reminder, we will run this command at the start of each lab, but only when we are inside a lab folder.
poetry shell
๐พ ๐ฌ Exiting the poetry shellWhen you want to exit the shell, you can type
exit
or^D
๐ป Take a look at the files inside with:
ls
example.py
modulo.py
pattern.py
user_input.py
extension_rainbow.py
[2] Writing Conditionals #
๐ป
Run the file example.py
to see this conditional statement in actions.
python example.py
๐ป Open the code:
code example.py
๐ป Experiment with changing the numbers in the conditional statements. Then run your code to see how your changes affect what it prints.
[3] Conditionals: User Input #
Now, let’s explore how we can use conditionals in conjunction with user input.
๐ป Start by running the file:
user_input.py
. One way to use conditionals in our drawings is to use them to respond to user input.
Try asking the program to draw a square.
python user_input.py
code user_input.py
# user_input.py
from turtle import *
for i in range(3):
drawing = input("What would you like me to draw? ")
size = int(input("How big should I draw it? "))
if drawing == "square":
for i in range(4):
forward(size)
right(90)
elif drawing == "circle":
circle(size)
else:
print("Sorry, I don't know how to draw that...")
clear()
This program has a lot of potential, but so far it can only generate two drawings.
๐ป Add at least two more branches to the conditional so that the program can draw more than just a square and a circle.
An elif
statement will probably be useful here.
For example, your program could also draw a triangle and a pentagon.
[4] Modulo #
Python has many operators that allow you to perform calculations with values. You’ve probably
seen and used the basic ones like +
(add), -
(subtract), *
(multiply), and /
(divide).
However, Python has some other less common operators that can be really helpful.
One such operator is the modulo operator (%
). This operator takes two values, divides them, and returns the remainder of the division.
For example:
5/2 has a remainder of 1
5%2
= 1
๐ป
Open and run the file modulo.py
to experiment with the modulo
operator. Does it output what you expected?
print(5%2)
print(3%3)
print(6%2)
print(9%2)
Even/Odd Pattern #
Conditionals can also be paired with the modulo operator to cause your code to run in repeated patterns.
๐ป Start by running
pattern.py
. You should see a series of red boxes.
python pattern.py
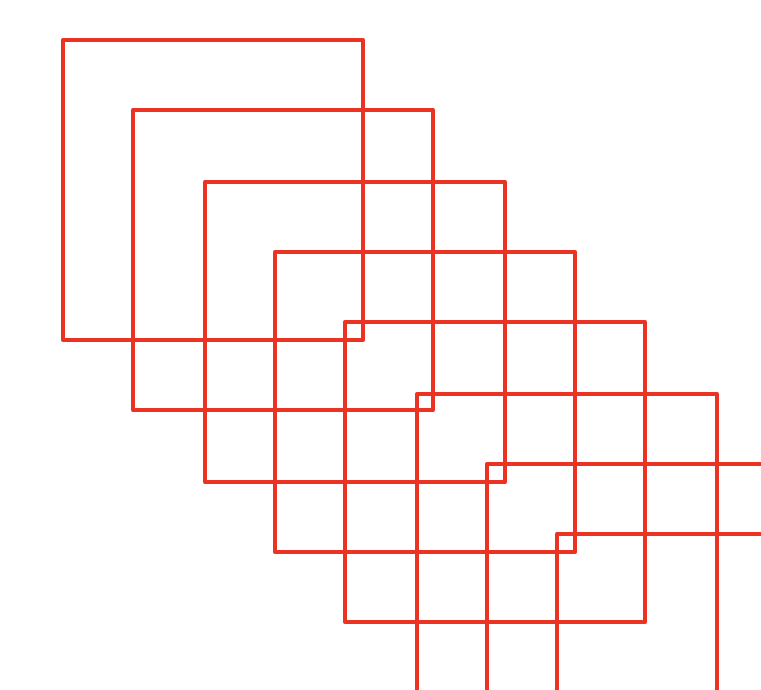
One common use of the
modulo
operator, is to determine if a number is even or odd. Use that in conjunction with conditional statements to create an alternating color pattern.
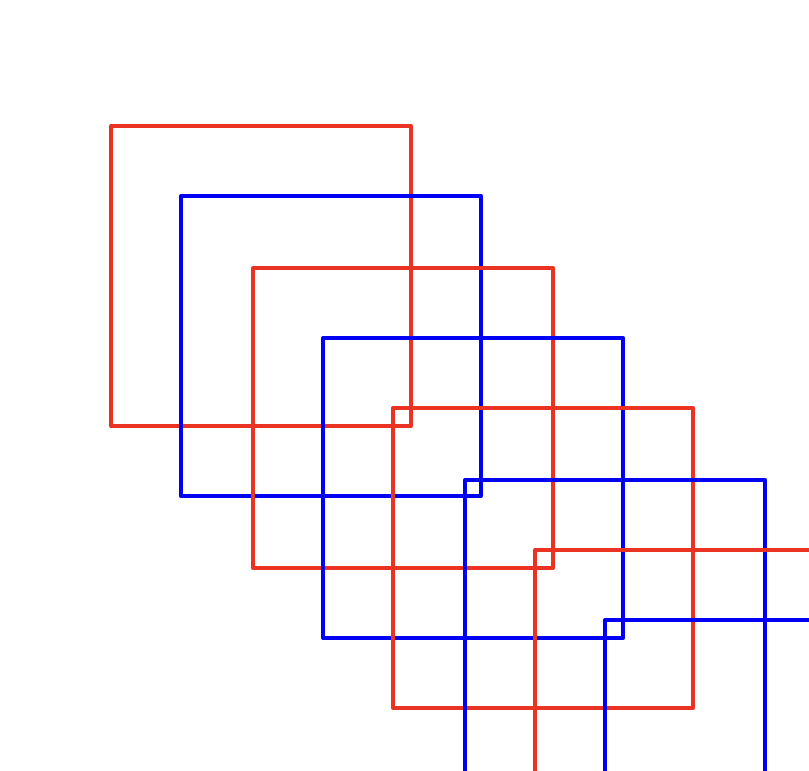
[5] Deliverables #
โกโจOnce you’ve successfully completed the pattern be sure to fill out this Google form.
โ๏ธ Add a piece of your code and a screenshot to the
CS Year 1 Code Log
in your Google Drive. Add a comment. You can:
- ask a question about your code
- write a tip to your future self
- explain a bug you fixed in your code
- describe a piece of code you are proud of
[6] Extension: Rainbow #
๐ป Start by running
extension_rainbow.py
. You should see a series of colored boxes in a rainbow - very similar to the previous program.
python extension_rainbow.py
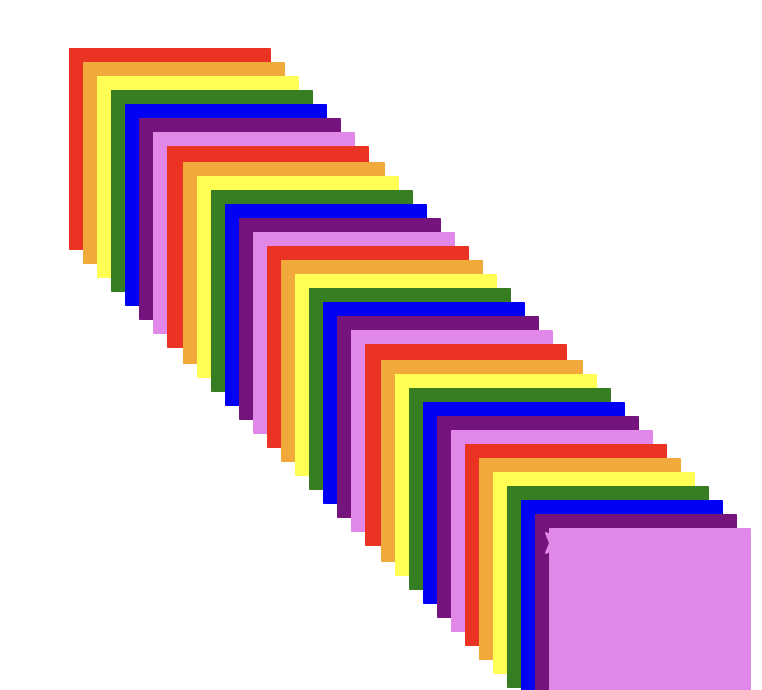
๐ป Open up the code and see how it works
extension_rainbow.py
. As you can see the code is over 80 lines long!
code extension_rainbow.py
Although this code is really really long, there is a pattern to the rainbow that we could use to simplify the code!
๐ป Simplify this code using conditional statements and the modulo operator. Your file should be less than 40 lines of code when successfully simplified.