Riddle Server #
In this lab we are going to learn how the riddle server is made using Banjo.
π Open the Banjo documentation: the-isf-academy.github.io/banjo_docs/
[0] Set Up #
π» Download Mac app of HTTPIE: httpie.io/download. We need to download the app to make local HTTP requests for testing purposes. In this lab, you will run a locally hosted riddle server on your laptop using Banjo.
π» Start by going into the unit folder and the lab.
Remember to replace yourgithubusername
with your actual GitHub username.
cd ~/desktop/making_with_code/unit03_networking/
cd lab_banjo_yourgithubusername
poetry update
poetry shell
[1] Local Riddle Server #
You are each able to run a locally hosted riddle server on your laptop using Banjo.
Starting the Server #
π» Now, let's start your local server.
We use --debug
to provide more information when in the development stage.
banjo --debug
No changes detected in apps 'app', 'banjo'
Operations to perform:
Apply all migrations: admin, app, auth, contenttypes, sessions
Running migrations:
No migrations to apply.
No changes detected in apps 'banjo', 'app'
Operations to perform:
Apply all migrations: admin, app, auth, contenttypes, sessions
Running migrations:
No migrations to apply.
Performing system checks...
System check identified no issues (0 silenced).
September 16, 2022 - 02:40:21
Django version 4.1.1, using settings 'banjo.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CONTROL-C.
Accessing the Server #
π» You can now visit this server in your web browser, just as you did with the riddler server hosted on the internet: 127.0.0.1:8000/riddle/all
π» Open the HTTPie
desktop app to send the same GET
request to /all
.
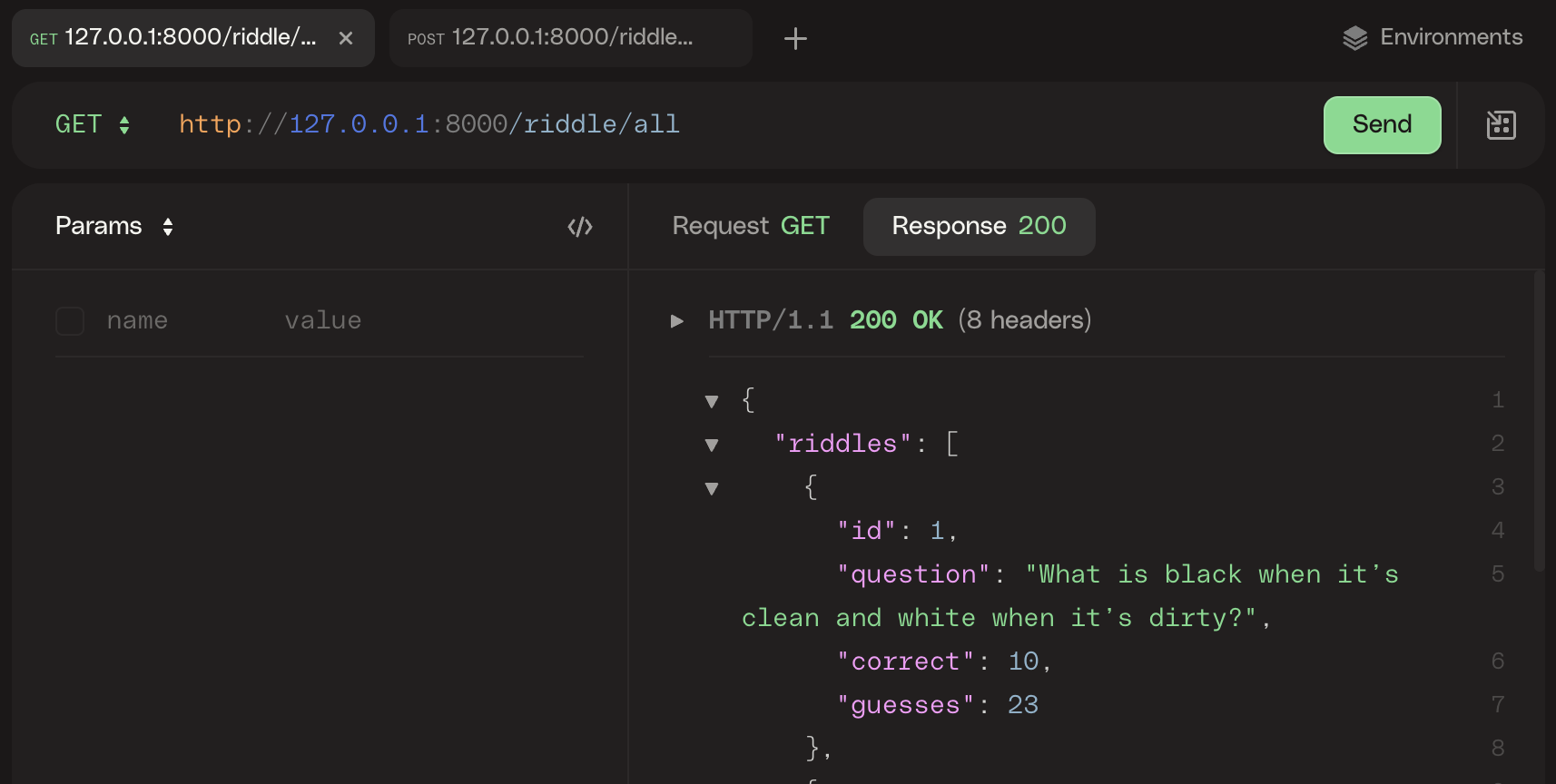
[16/Sep/2024 02:44:20] "GET /riddle/all HTTP/1.1" 200 1069
π» Send a POST
request to the /new
endpoint.
Payload:
question
(str)answer
(str)
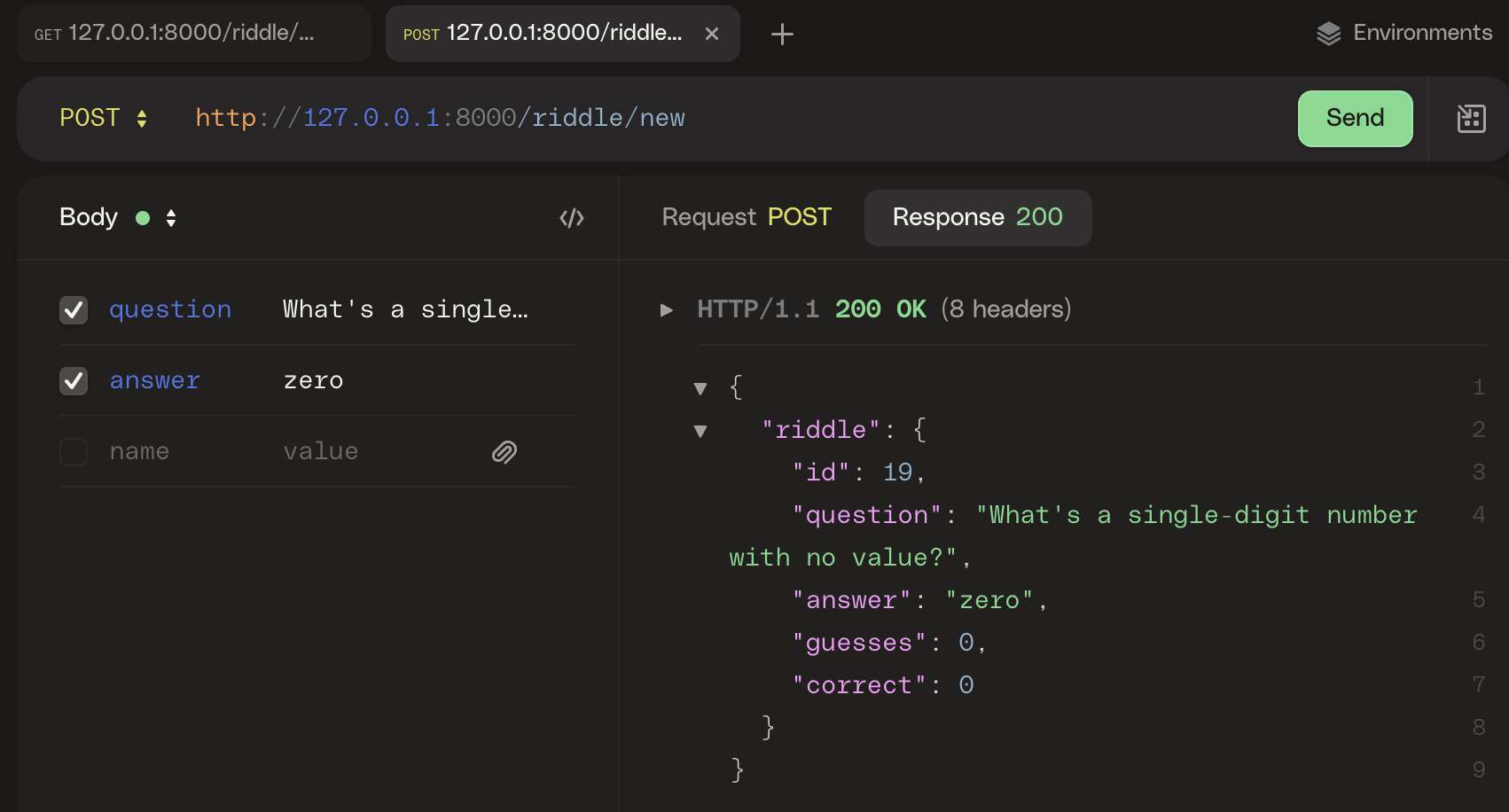
[01/Sep/2024 20:56:22] "POST /riddle/new?id=1 HTTP/1.1" 200 127
Your version of the riddle server only has the 2 endpoints:
/riddle/all
/riddle/new
β CHECKPOINT:π» Explore both endpoints via the HTTPie desktop app and be sure to successfully:
- view all riddles without the answers
- create a new riddle
[2] What is Banjo? #
This server is written using Banjo, a wrapper for Django. It allows users to quickly create models with a persistant database and API.
π Banjo apps must have an app
folder. Within the app folder must be two files: models.py
, views.py
. The database.sqlite
file is created when the server is first started. It is stored at the same level as app
. Here is an example file structure:
lab_banjo
|
βββapp
| βββmodels.py
| βββviews.py
βββdatabase.sqlite
models.py
- This defines what the database will look like. Models are a lot like classes. Just as a class has properties, a model has fields. When defining a model’s fields, you must specify the data type.views.py
- The views are where you define the API functionality. Here is where you decide what theendpoints
are and the type ofHTTP
request it will require.
π In this lab, we are going to be primarily focused on the views.py
file.
[3] Writing Routes #
In this lab, you will build out the functionality of the Riddle server. Currently, your file only has riddle/all
and riddle/new
.
It is up to you to add the following endpoints:
riddle/one
riddle/guess
riddle/difficulty
riddle/random
π» Start by opening up the primary folder:
/app
code app
πΎ π¬Open a new terminal tab using
β + T
.
You can have your server running in one tab, and use the other tab to access your filesystem.
π»
Open the views.py
file. Here is where you will write the additional endpoints.
π Open the Banjo Views Documentation:
the-isf-academy.github.io/banjo_docs/ You will need to reference this and the exisiting routes in views.py
.
riddle/one #
π»
Write the riddle/one
endpoint.
- HTTP method:
get
- Payload/args:
id
- Return: a single
Riddle
with thequestion
,guesses
, andcorrect
properties
π€ Which method
in the Riddle
model
could be useful?
β CHECKPOINT:π» Test the
riddle/one
endpoint in theHTTPie desktop app
http://127.0.0.1:8000/riddle/one
βοΈ It should return
json
like:{ "riddle": { "id": 1, "question": "Iβm light as a feather, yet the strongest person canβt hold me for five minutes. What am I?", "guesses": 43, "correct": 1 } }
riddle/guess #
π»
Write the riddle/guess
endpoint.
- HTTP method:
post
- Payload/args:
id
andguess
- Return:
- if the guess was correct
- message telling the user they were correct
- a single
Riddle
with theid
,question
,guesses
,correct
, andanswer
properties
- if the guess was incorrect
- message telling the user they were incorrect
- a single
Riddle
with theid
,question
,guesses
, andcorrect
, properties
- if the guess was correct
π€ Which method
in the Riddle
model
could be useful?
β CHECKPOINT:π» Test the
riddle/guess
endpoint in theHTTPie desktop app
http://127.0.0.1:8000/riddle/guess
βοΈ It should return
json
like:{ "riddle": { "id": 1, "question": "Iβm light as a feather, yet the strongest person canβt hold me for five minutes. What am I?", "guesses": 44, "correct": false } }
riddle/difficulty #
π»
Write the riddle/difficulty
endpoint.
- HTTP method:
get
- Payload/args:
id
- Return:
- a single
Riddle
with theid
,question
, anddifficulty
properties
- a single
π€ Which method
in the Riddle
model
could be useful?
β CHECKPOINT:π» Test the
riddle/difficulty
endpoint in theHTTPie desktop app
http://127.0.0.1:8000/riddle/difficulty
βοΈ It should return
json
like:{ "riddle": { "id": 1, "question": "Iβm light as a feather, yet the strongest person canβt hold me for five minutes. What am I?", "difficulty": 0.9555555555555556 } }
riddle/random #
π»
Write the riddle/random
endpoint.
- HTTP method:
get
- Payload/args: none
- Return: a single
Riddle
with theid
,question
,correct
, andguess
properties
π€ Which query method may be useful? Be sure to reference the Banjo documentation.
β CHECKPOINT:π» Test the
riddle/random
endpoint in theHTTPie desktop app
http://127.0.0.1:8000/riddle/random
βοΈ It should return
json
like:{ "riddle": { "id": 6, "question": "The more you take, the more you leave behind. What am I?", "guesses": 4, "correct": 0 } }
[4] Deliverables #
β‘β¨Once you’ve successfully completed the worksheet be sure to fill out this Google form.
π» Push your work to Github:
- git status
- git add -A
- git status
- git commit -m “describe your code and your process here”
be sure to customize this message, do not copy and paste this line
- git push
[5] Extensions #
View Solution #
Currently, there’s no way to see the answer unless you correctly guess the riddle.
π» Write an endpoint that has the ability to view the solution of a given Riddle.
- an existing
Riddle
class may be useful
It should return JSON
that looks something like:
{
"riddle": [
{
"correct": 0,
"guesses": 3,
"id": 1,
"question": "It goes up and down the stairs without moving.",
"answer": "A carpet"
}
]
}
Change Question and Answer #
π» Write an endpoint that has the ability to change the question a riddle.
- *It may be helpful to write a new method
change_question()
Method | URL | Required Payload | Action |
---|---|---|---|
POST | /change/question | new_question | Returns thee riddle with the new answer |
π» Write an endpoint that has the ability to change the answer a riddle.
- *It may be helpful to write a new method
change_answer()
Method | URL | Required Payload | Action |
---|---|---|---|
POST | /change/answer | new_answer | Returns the riddle with the new answer |
Difficulty Level Endpoint #
Because we track difficulty
, it would be nice if we could GET
a list of riddles of easy
, medium,
or hard
difficulty.
π» Write an endpoint returns riddles within a difficulty category
- a Riddle with a difficulty of 1 is impossibly hard, while a Riddle with a difficulty of 0 is easy–everyone gets it right!
Method | URL | Required Payload | Action |
---|---|---|---|
GET | /all/difficulty | level (easy, medium, or hard) | Returns a list of riddles of the appropriate difficulty level |
βοΈ It should return json
like:
{
"difficulty_level": "hard",
"riddles": [
{
"correct": 1,
"guesses": 44,
"id": 1,
"question": "Iβm light as a feather, yet the strongest person canβt hold me for five minutes. What am I?",
"difficulty": 0.9555555555555556
},
{
"correct": 4,
"guesses": 9,
"id": 2,
"question": "What comes down but never goes up?",
"difficulty": 0.875
}
]
}
Like field #
What if we want to know which riddles people like? For this, we will have to add allow users to like a riddle.
Let’s make the Riddle
keep track of likes
. You will need to edit models.py
π»
Let’s make the Riddle
keep track of likes
. You will need to edit models.py
You will need add a new field and write a new method.
π» Add a new endpoint for you to get all Riddle objects of a category
Method | URL | Required Payload | Action |
---|---|---|---|
POST | /one/like | id | Returns a single riddle with the likes |
{
"riddle": {
"id": 1,
"question": "Iβm light as a feather, yet the strongest person canβt hold me for five minutes. What am I?",
"guesses": 43,
"correct": 1,
"likes": 10
}
}